Quick guide for Zoom Integration and Create Meeting using Python
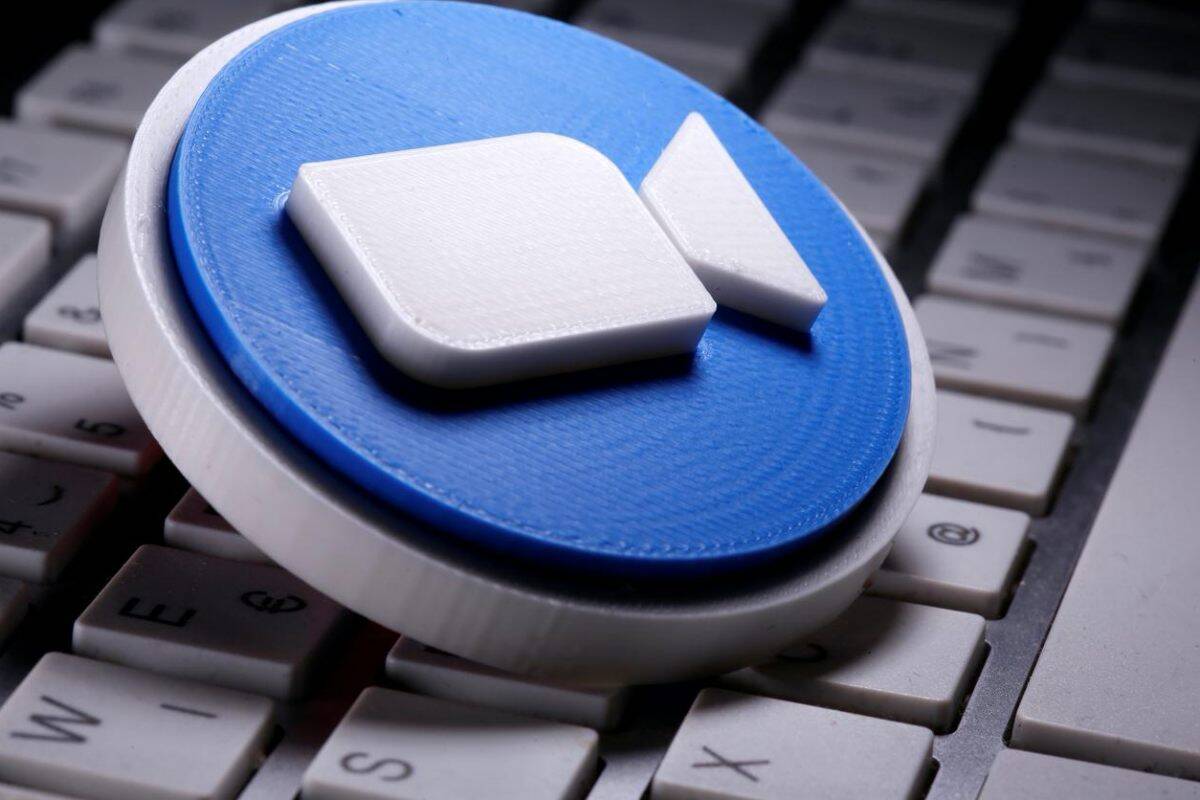
Step 1 : Create Oauth App and get installation URL for authorization
- - Visit https://marketplace.zoom.us/
- - Click `Develop` dropdown and then `Build App`
- - Create Oauth App
- - Provide App Details like name, app type
- - Add Redirect URL (Eg. URL to which Zoom service will return code https://someurl.com)
- - Add API Scopes
- - Provide App description and developer information
- - Click Continue
- - After finishing you will get a Zoom installation URL,
Eg. https://zoom.us/oauth/authorize?response_type=code
&client_id=<ClientId>
&redirect_uri=https%3A%2F%2Fsomeurl.com
(Note: This URL is used to ask User to authorize access to app for Zoom information)
Step 2 : User provides authorization for zoom access using installation url
- Once authorized, it will be routed to redirect URL with a code
Eg. https://someurl.com/api/auth?code=fg123afsag123asfasd123afasd
Step 3 : Get Access Token
- Before getting the access token, generate authorization code using clientID and clientSecret.
- Encode `ClientID:ClientSecret` to base64. Client Id and Client Secret are colon separated. You can use https://www.base64encode.org/ for encoding.
Eg. Q2xpZW50SUQ6Q2xpZW50U2VjcmV0 (After encoding) - Encoded Authorization Key - This key will be required in the Headers for all the API requests further
Now, to get Access Token for a client
Example Request:
POST
https://zoom.us/oauth/token?grant_type=authorization_code&code=<code>&redirect_uri=<redirect_url>
Request Headers:
{
"Authorization": "Basic <Encoded Authorization Key>"
}
If successful, the response body will be a JSON representation of the user’s access token:
{
"access_token": "<Access Token Provided By Zoom>",
"token_type": "bearer",
"refresh_token": "<Refresh Token used to refresh access token>",
"expires_in": 3599,
"scope": "user:read:admin"
}
This access token can now be used to make requests to the Zoom API. Access tokens expire after 1 hour.
Step 4 : Get User Information using Access token - https://api.zoom.us/v2/users/me
GET
https://api.zoom.us/v2/users/me
Request Headers:
{
"Authorization": "Basic <Encoded Authorization Key>"
}
If successful, the response body will be a JSON representation of the user’s information:
{
"id": "KdYKjnimT4KPd8FFgQt9FQ",
"first_name": "Jane",
"last_name": "Dev",
"email": "jane.dev@email.com",
"type": 2,
"role_name": "Owner",
"pmi": 1234567890,
"use_pmi": false,
"vanity_url": "https://janedevinc.zoom.us/my/janedev",
"personal_meeting_url": "https://janedevinc.zoom.us/j/1234567890",
"timezone": "America/Denver",
"verified": 1,
"dept": "",
"created_at": "2019-04-05T15:24:32Z",
"last_login_time": "2019-12-16T18:02:48Z",
"last_client_version": "4.6.12611.1124(mac)",
"pic_url": "https://janedev.zoom.us/p/KdYKjnimFR5Td8KKdQt9FQ/19f6430f-ca72-4154-8998-ede6be4542c7-837",
"host_key": "533895",
"jid": "kdykjnimt4kpd8kkdqt9fq@xmpp.zoom.us",
"group_ids": [],
"im_group_ids": [
"3NXCD9VFTCOUH8LD-QciGw"
],
"account_id": "gVcjZnYYRLDbb_MfgHuaxg",
"language": "en-US",
"phone_country": "US",
"phone_number": "+1 1234567891",
"status": "active"
}
Step 5 : Now to create meeting on Client Profile using Python
- Install zoomus
pip install zoomus
Follow below code for creating meeting
import json
from zoomus import ZoomClient
from datetime import datetime
# connect to Zoom Client
client = ZoomClient('ClientID', 'ClientSecret')
# Create Meeting
client.meeting.create(user_id='UserId from above API response', topic='My New Meeting', start_time=datetime.now())
# Find if Meeting got created
user_list = json.loads(client.user.list().content)
for user in user_list['users']:
user_id = user['id']
meetings = client.meeting.list(user_id=user_id).content
print(json.loads(meetings))
For more API usage information, visit
https://marketplace.zoom.us/docs/api-reference/zoom-api
For Python Zoomus Library, visit
https://github.com/prschmid/zoomus
Powered by Froala Editor